Découvrez nos ressources
Profitez d’un accès illimité à des articles de qualité sur les dernières tendances en éducation et en entreprise.
Participez à des programmes de formation
Mettez à jour vos compétences et améliorez votre expertise grâce à nos formations pratiques et interactives.
Rejoignez notre communauté
Obtenez des conseils d’experts et échangez avec d’autres professionnels passionnés par le développement en entreprise.
Découvrez Qui Nous Sommes
Éducation Expert est votre destination de prédilection pour tout ce qui concerne l’entreprise et le développement professionnel.
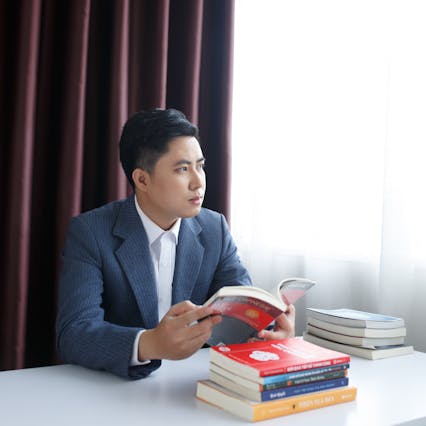
Nourrissez votre esprit avec notre contenu exclusif sur l’actualité, la formation et le développement professionnel.
Renouveler vos connaissances
Approfondissez et élargissez vos compétences grâce à notre contenu éducatif à jour.
Faire des choix éclairés
Nous fournissons des ressources claires et fiables pour vous aider à prendre des décisions avisées en matière de développement professionnel.
Explorez nos ressources
Articles spécialisés
Nos articles couvrent une variété de sujets pertinents pour les professionnels et les entreprises, allant de l’actualité à la formation en passant par les stratégies de marketing et bien plus encore.
Développement de carrière
Découvrez nos programmes de formation approfondis conçus pour stimuler votre croissance professionnelle et vous aider à atteindre vos objectifs professionnels.
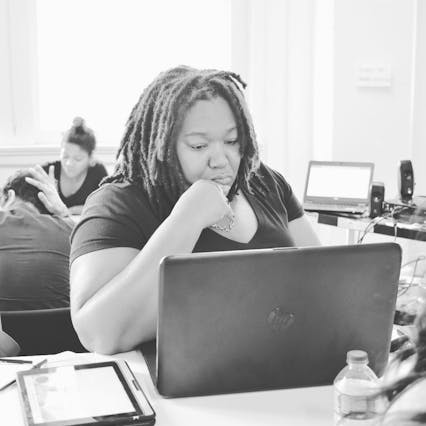
Chiffres clés
Découvrez notre impact et notre expertise en développement professionnel.
Actu
250K
Business
500K
Formation
1M
Marketing
750K
Nous proposons une variété unique de services pour vous aider à atteindre vos objectifs.
Grâce à nos formations de haute qualité et à notre expertise en marketing, gestion et autres domaines clés, vous ferez la différence dans votre secteur.
Formations engageantes
Découvrez nos formations interactives conçues pour développer vos compétences et vos connaissances en entreprise.
Stratégies de marketing éprouvées
Réalisez votre potentiel avec nos techniques de marketing éprouvées pour promouvoir votre entreprise et augmenter votre visibilité.
Expertise en gestion
Améliorez vos performances en tant que responsable en utilisant nos ressources spécialisées en matière de gestion et de leadership.
Consultation personnalisée
Notre équipe de professionnels vous fournira des conseils personnalisés pour vous aider à réussir dans votre domaine.
Articles récents
Restez à jour sur les dernières actualités et tendances de l’industrie grâce à notre blog d’entreprise.
Guide Ultime : Élaborer une Stratégie de Marketing de Marque Gagnante
Comprendre l’importance d’une stratégie de marketing de marque Une stratégie de marketing de marque réussie[…]
L’Impact Crucial de la Psychologie du Travail sur le Leadership Efficace
Les Principes Clés de la Psychologie du Travail La psychologie du travail joue un rôle[…]
Maîtrise des Risques Environnementaux dans l’Industrie Chimique : Techniques Incontournables et Innovantes
Techniques incontournables pour la gestion des risques environnementaux dans l’industrie chimique La gestion des risques[…]
Identifier et Transformer une Culture d’Entreprise Toxique : Guide Complet
Comprendre la culture d’entreprise toxique Une culture d’entreprise toxique se caractérise par des pratiques préjudiciables[…]
Gérer le Stress au Travail : L’Art de la Pause Efficace
Importance des Pauses pour Gérer le Stress au Travail Dans le monde professionnel d’aujourd’hui, la[…]
Incorporer la réalité virtuelle et augmentée dans les programmes de formation numérique en entreprise
Les premières étapes pour intégrer la réalité virtuelle et augmentée dans la formation en entreprise[…]
Conception d’Emballage : Astuces pour Briller en Rayons et Attirer le Regard
Stratégies de Conception d’Emballage Dans l’univers compétitif du commerce, développer des stratégies d’emballage efficaces est[…]
Les Effets Surprenants des Rumeurs de Fusions-Acquisitions sur les Marchés Boursiers
Introduction aux rumeurs de fusions-acquisitions Les rumeurs de fusions-acquisitions sont des éléments fascinants, souvent chuchotés[…]
Le Statut d’Auto-Entrepreneur : La Solution Parfaite pour les Travailleurs Indépendants ?
Comprendre le Statut d’Auto-Entrepreneur Le statut auto-entrepreneur, une formule simplifiée de travail indépendant, se distingue[…]
L’Impact Stratégique du Conseil en Management pour un Développement Durable et Responsable
L’importance du Conseil en Management dans le Développement Durable Le Conseil en management joue un[…]
Guide Ultime du Marketing Digital pour Non-Experts : Démystifiez les Techniques Modernes
Introduction aux concepts fondamentaux du marketing digital Dans le monde moderne, l’introduction au marketing digital[…]
Comment la digitalisation peut-elle transformer votre modèle d’affaires?
Fondements de la digitalisation et impact sur les modèles d’affaires La digitalisation désigne l’intégration des[…]
L’Impact Essentiel des Services de Courrier sur la Logistique des Colis en Entreprise
L’importance des services de courrier dans la logistique des entreprises Dans le monde moderne des[…]
Comprendre les Implications Financières des Litiges Commerciaux : Décryptage et Stratégies
Comprendre les Litiges Commerciaux et leurs Implications Financières Dans le monde des affaires modernes, les[…]
Comment évaluer efficacement le ROI de votre stratégie sur les réseaux sociaux?
Comprendre le ROI des réseaux sociaux Le ROI des réseaux sociaux est un indicateur essentiel[…]
Maîtrisez L’Art de la Négociation : Formez-vous pour Devenir un Expert en Conviction et Persuasion
Les fondamentaux de la négociation et de la persuasion Comprendre les bases de la négociation[…]
La Gestion Stratégique : Clé de Voûte pour Surmonter les Défis Entrepreneuriaux
Introduction à la Gestion Stratégique Les concepts de gestion stratégique sont essentiels pour les entrepreneurs[…]
Les pièges fréquents de la gestion du temps : Comment les éviter et optimiser votre journée
Identifier les pièges courants de la gestion du temps Pour bien gérer son temps et[…]
Découvrez 6 Techniques Éprouvées de Gestion du Temps Apprises en Formation Professionnelle
Les Fondations de la Gestion du Temps Efficace en Milieu Professionnel Un pilier indispensable dans[…]
Comment fidéliser vos clients grâce à un service personnalisé?
Comprendre l’importance du service personnalisé pour la fidélisation client Le service personnalisé joue un rôle[…]
Convertir un CDD en CDI : Guide Ultime pour une Transition Réussie
Cadre légal de la conversion d’un CDD en CDI Naviguer dans le cadre légal de[…]